자바스크립트
1. 자료
자료형(type)
변수
연산자
단항
++ / -- / +/-
이항
거의 모든 연산자
삼항
? : (조건)
형변환 - 함수
숫자 - number(), parseInt(), parseFloat()
문자 - string()
불 - boolean()
집합자료형 - 배열((3)다차원)
* 길이(방개수)
사용자정의 자료형 : 객체(Object) -> Class
객체 중심의 프로그램 기법 - OOP(Object Oriented Programming)
사용자정의 자료형 - 프로그래머
내장객체 (무지많다) - 주어진다
라이브러리 - 주어진다
객체
item --(abstraction:추출)--> 객체(공통점) --(기술)--> 컴퓨터(class) -> 변수(instance)
객체 => 함수 - 기능 : Method
변수 - 속성 : Member variable
2. 제어
* 중첩
조건
if / switch / 삼항연산자
반복
for / for in / while / do ~ while
기타
break / continue
-----------------------------------------------------------------------------------------------------------------------
함수
선언적
function 함수명() {
내용;
}
익명 - 일급함수 : 함수의 정의를 변수값 취급할 수 있다
var 변수명 = function() {
내용;
};
=> 함수 호출
=> 사용자 정의 함수 / 내장 함수(O)
3. 객체
사용자 정의 객체
내장 객체
자료형 - 기본 자료형과 관련된 객체
DOM - Document Object Model(HTML)
BOM - Browser Object Model(Browser 제어)
외부 라이브러리
js.58 빈 객체의 선언
<!doctype html />
<html lang="ko">
<head>
<meta charset="utf-8"/>
<style type="text/css">
</style>
<script type="text/javascript">
</script>
</head>
<body>
<script type="text/javascript">
// 빈객체의 선언
var product1 = {};
// 멤버 변수, 메서드
var product2 = {
name: '7D 건조 망고',
type: '당절임',
component: '망고, 설탕, 기타',
region: '필리핀'
};
// 배열[값] - 인덱스
// 객체[값] - 키
console.log(product2['name']);
console.log(product2['type']);
// . : 객체 참조 연산자
console.log(product2.name);
console.log(product2.type);
product2.name = '3D 건조 딸기';
console.log(product2['name']);
</script>
</body>
</html>


js.59 객체의 속성(num과 product 값 수정시 반영 정도)
<!doctype html />
<html lang="ko">
<head>
<meta charset="utf-8"/>
<style type="text/css">
</style>
<script type="text/javascript">
</script>
</head>
<body>
<script type="text/javascript">
var product1 = {
name: '7D 건조 망고',
type: '당절임',
component: '망고, 설탕, 기타',
region: '필리핀'
};
var product2 = product1;
console.log(product1['name']);
console.log(product2['name']);
product1.name = '3D 건조 딸기';
console.log(product1['name']);
console.log(product2['name']);
var num1 = 10;
var num2 = num1;
console.log("num1 : " + num1);
console.log("num2 : " + num2);
num1 = 20;
console.log("num1 : " + num1);
console.log("num2 : " + num2);
</script>
</body>
</html>



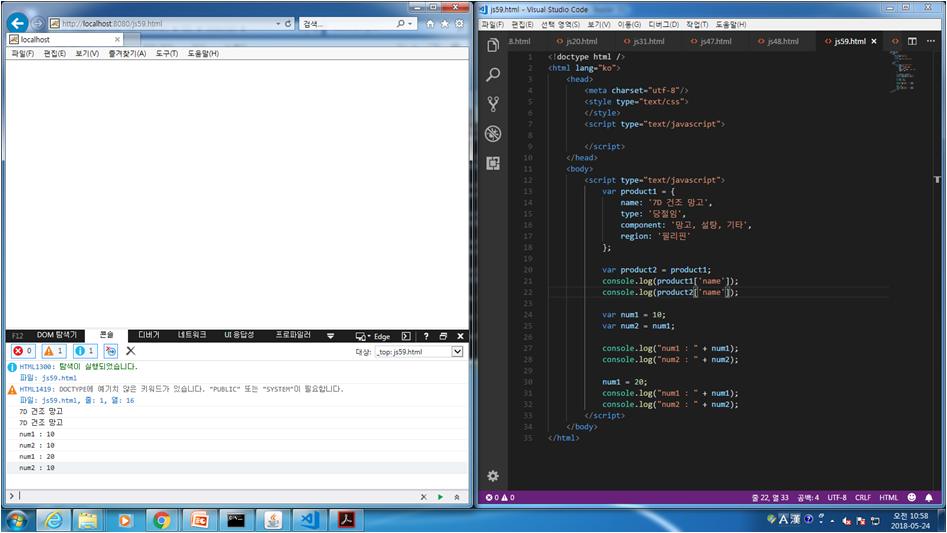
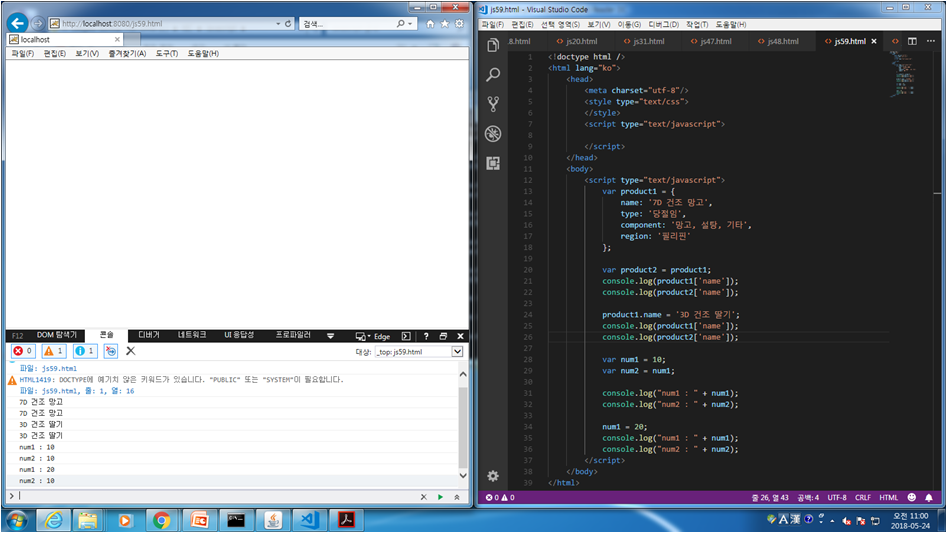
js.60 in 추가
<!doctype html />
<html lang="ko">
<head>
<meta charset="utf-8"/>
<style type="text/css">
</style>
<script type="text/javascript">
</script>
</head>
<body>
<script type="text/javascript">
// 멤버변수(Property / Field)
var product1 = {
name: '7D 건조 망고',
type: '당절임',
components: '망고, 설탕, 기타',
region: '필리핀',
// 메서드
eat: function() {
console.log(name + '맛있게 먹는다');
// this - 자기참조
console.log(this.name + '맛있게 먹는다');
this.test();
},
test: function() {
console.log('테스트 한다');
}
};
product1.eat();
product1.test();
var output = '';
for(var key in product1) {
output += key + ':' + product1[key] + '\n';
}
console.log(output);
</script>
</body>
</html>

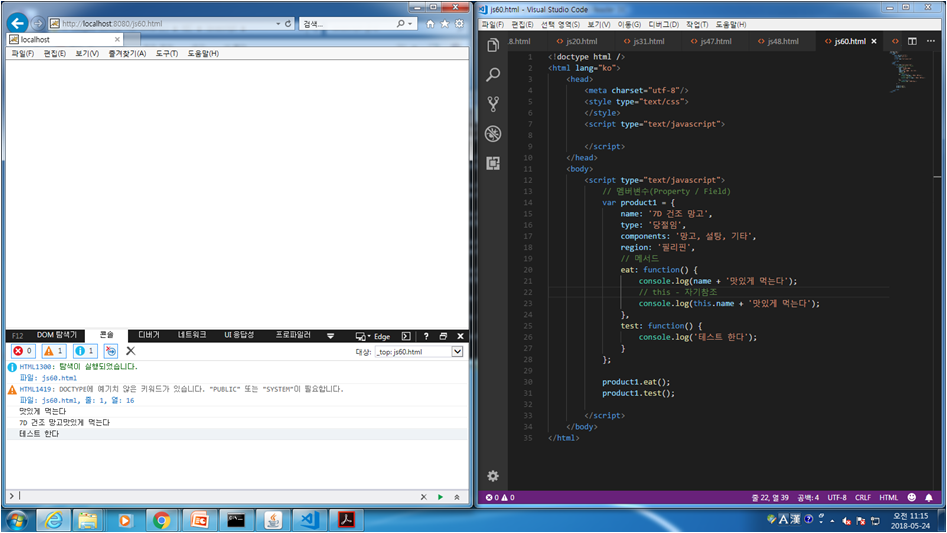



js.61 with 추가
<!doctype html />
<html lang="ko">
<head>
<meta charset="utf-8"/>
<style type="text/css">
</style>
<script type="text/javascript">
</script>
</head>
<body>
<script type="text/javascript">
var student = {
name: '홍길동',
kor: 92,
mat: 98,
eng: 96,
sci: 98
}
// in : 내부적인 필드명 존재를 확인
console.log(name in student);
console.log('name' in student);
console.log(student.name);
console.log(student.kor);
with(student) {
console.log(name);
console.log(mat);
}
</script>
</body>
</html>



js.62 동적 추가 / 삭제
<!doctype html />
<html lang="ko">
<head>
<meta charset="utf-8"/>
<style type="text/css">
</style>
<script type="text/javascript">
</script>
</head>
<body>
<script type="text/javascript">
var student = {};
// 동적 추가
student.name = '박문수';
student.hobby = '악기';
var output = '';
for(var key in student) {
output += key + ':' + student[key] + '\n';
}
console.log(output);
</script>
</body>
</html>
-----------------------------------------------------------------------------------------------------------------------
<!doctype html />
<html lang="ko">
<head>
<meta charset="utf-8"/>
<style type="text/css">
</style>
<script type="text/javascript">
</script>
</head>
<body>
<script type="text/javascript">
var student = {};
// 동적 추가
student.name = '박문수';
student.hobby = '악기';
student.test = function() {
console.log('test() 호출');
}
// toString - 객체 내부의 값을 확인할 때
student.toString = function() {
var output = '';
for(var key in this) {
if (key != 'toString') {
output += key + ':' + this[key] + '\n';
}
}
return output;
}
console.log(student.toString());
// 삭제
delete(student.name);
console.log(student.toString());
</script>
</body>
</html>



js.63 함수를 이용한 객체 생성
<!doctype html />
<html lang="ko">
<head>
<meta charset="utf-8"/>
<style type="text/css">
</style>
<script type="text/javascript">
</script>
</head>
<body>
<script type="text/javascript">
// 함수를 이용한 객체 생성
function makeStudent(name, kor, mat) {
var obj = {
m_name: name,
m_kor: kor,
m_mat: mat,
getSum:function() {
return this.m_kor + this.m_mat;
},
getAverage:function() {
return this.getSum() / 2;
}
}
return obj;
}
var stu1 = makeStudent('홍길동', 96, 98);
var stu2 = makeStudent('박문수', 94, 90);
console.log(stu1);
console.log(stu2);
console.log(stu1.m_name + ' : ' + stu1.getSum());
console.log(stu2.m_name + ' : ' + stu2.getSum());
console.log(stu1.m_name + ' : ' + stu1.getAverage());
console.log(stu2.m_name + ' : ' + stu2.getAverage());
</script>
</body>
</html>


js.64 생성자 함수
<!doctype html />
<html lang="ko">
<head>
<meta charset="utf-8"/>
<style type="text/css">
</style>
<script type="text/javascript">
</script>
</head>
<body>
<script type="text/javascript">
// 함수를 이용한 객체 생성
function Student(name, kor, mat) {
this.name = name;
this.kor = kor;
this.mat = mat;
this.getSum = function() {
return this.kor + this.mat;
};
this.getAverage = function() {
return this.getSum() / 4;
};
}
// new - 객체 생성 연산자
// Student - 생성자 함수(constructor)
// stu1, stu2 - 객체 변수(참조변수), instance
var stu1 = new Student('홍길동', 96, 98);
var stu2 = new Student('박문수', 90, 94);
console.log(stu1.name + ' : ' + stu1.getSum());
console.log(stu2.name + ' : ' + stu2.getSum());
</script>
</body>
</html>

js.65 프로토타입
<!doctype html />
<html lang="ko">
<head>
<meta charset="utf-8"/>
<style type="text/css">
</style>
<script type="text/javascript">
</script>
</head>
<body>
<script type="text/javascript">
// 함수를 이용한 객체 생성
function Student(name, kor, mat) {
this.name = name;
this.kor = kor;
this.mat = mat;
// 메서드(X)
}
Student.prototype.getSum=function() {
return this.kor + this.mat;
};
Student.prototype.getAverage=function() {
return this.getSum() / 4;
};
var stu1 = new Student('홍길동', 96, 98);
var stu2 = new Student('박문수', 90, 94);
console.log(stu1.name + ' : ' + stu1.getSum());
console.log(stu2.name + ' : ' + stu2.getSum());
console.log(stu1);
</script>
</body>
</html>


js.66 String 객체
<!doctype html />
<html lang="ko">
<head>
<meta charset="utf-8"/>
<style type="text/css">
</style>
<script type="text/javascript">
</script>
</head>
<body>
<script type="text/javascript">
var str1 = 'Hello String';
var str2 = new String('Hello String');
console.log(str1);
console.log(str2);
console.log(str1 + "!!");
console.log(str2 + "!!");
</script>
</body>
</html>

js.67 기본 속성과 메서드
<!doctype html />
<html lang="ko">
<head>
<meta charset="utf-8"/>
<style type="text/css">
</style>
<script type="text/javascript">
</script>
</head>
<body>
<script type="text/javascript">
var str1 = 'Hello String 123';
// 문자열 길이
console.log(str1.length);
// 문자 추출
var char1 = str1.charAt(0);
console.log(char1);
var char1 = str1.charAt(6);
console.log(char1);
console.log(str1[0]);
// 맨 마지막 문자
console.log(str1.charAt(str1.length - 1));
// 문자열 추출
// substr(시작위치, 갯수);
//var substr1 = str1.substr(6, 2);
//var substr1 = str1.substr(6);
// substring(시작위치, 끝날위치);
var substr1 = str1.substring(6, 11);
// slice
console.log(substr1);
// 문자 / 문자열의 위치 검색
var idx1 = str1.indexOf('l');
var idx2 = str1.lastIndexOf('l');
console.log(idx1);
console.log(idx2);
</script>
</body>
</html>

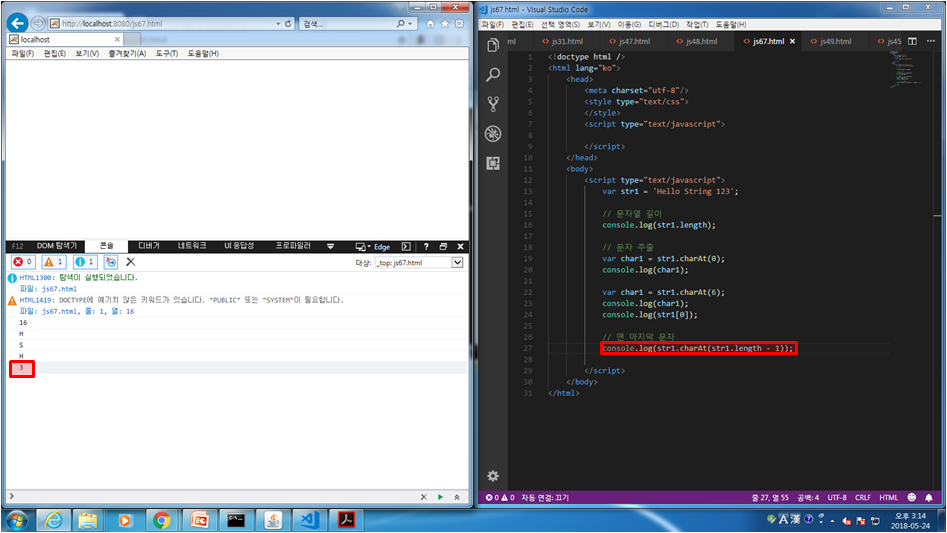




js.68 맨 앞글자만 대문자로
<!doctype html />
<html lang="ko">
<head>
<meta charset="utf-8"/>
<style type="text/css">
</style>
<script type="text/javascript">
</script>
</head>
<body>
<script type="text/javascript">
var str1 = 'hong gil dong';
var words = str1.split(' ');
var sentence = '';
//console.log(words[0]);
for(var i in words) {
//console.log(words[i]);
// 첫글자(substr(0, 1)) + 나머지(substr(1))
//console.log(words[i].substr(0, 1).toUpperCase()
//+ words[i].substr(1));
sentence += words[i].substr(0, 1).toUpperCase() + words[i].substr(1) + ' ';
}
console.log(sentence);
</script>
</body>
</html>
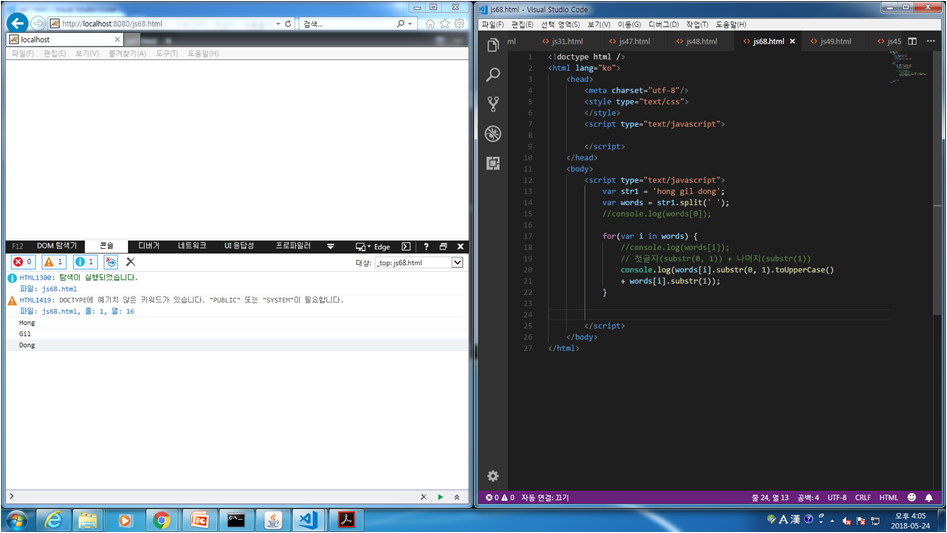
js.69 주민등록번호 판별기
<!doctype html />
<html lang="ko">
<head>
<meta charset="utf-8"/>
<style type="text/css">
</style>
<script type="text/javascript">
</script>
</head>
<body>
<script type="text/javascript">
var checkjumin="880518-1237611";
var jum1 = checkjumin.substring(0, 6);
var jum2 = checkjumin.substring(7, 14);
if((jum1.length==6)&&(jum2.length==7)) {
var jum = jum1 + jum2;
a=new Array(13);
for(var i=0; i<=13; i++) {
a[i]=parseInt(jum.charAt(i));
}
var ck = 11-(((a[0]*2)+(a[1]*3)+a[2]*4+a[3]*5+a[4]*6+a[5]*7+a[6]*8+a[7]*9+a[8]*2+a[9]*3+a[10]*4+a[11]*5)%11);
if(ck>10) {
ck-=10;
}
if(ck==a[12]) {
console.log('맞음');
}
else {
console.log('틀림');
}
}
else {
console.log('주민등록번호 13자리를 다시 입력하여 주세요')
}
</script>
</body>
</html>

---------------------------------------------------------------------------------------------------------
강사님이 한거
js.69_1
<!doctype html />
<html lang="ko">
<head>
<meta charset="utf-8"/>
<style type="text/css">
</style>
<script type="text/javascript">
</script>
</head>
<body>
<script type="text/javascript">
var strJumin="880518-1237611";
// - 제거
strJumin = strJumin.replace('-', '');
console.log(strJumin);
// 한 글자씩 분리
// 문자(X) / 문자열(O)
var str1 = parseInt(strJumin.substring(0,1))*2;
var str2 = parseInt(strJumin.substring(1,2))*3;
var str3 = parseInt(strJumin.substring(2,3))*4;
var str4 = parseInt(strJumin.substring(3,4))*5;
var str5 = parseInt(strJumin.substring(4,5))*6;
var str6 = parseInt(strJumin.substring(5,6))*7;
var str7 = parseInt(strJumin.substring(6,7))*8;
var str8 = parseInt(strJumin.substring(7,8))*9;
var str9 = parseInt(strJumin.substring(8,9))*2;
var str10 = parseInt(strJumin.substring(9,10))*3;
var str11 = parseInt(strJumin.substring(10,11))*4;
var str12 = parseInt(strJumin.substring(11,12))*5;
// checkbit
var str13 = parseInt(strJumin.substring(12,13));
console.log(str1);
console.log(str13);
var sum = str1 + str2 + str3 + str4 + str5 +str6 + str7
+ str8 + str9 + str10 + str11 + str12;
var result = (11 - (sum % 11))%10;
console.log(result);
console.log(str13);
</script>
</body>
</html>
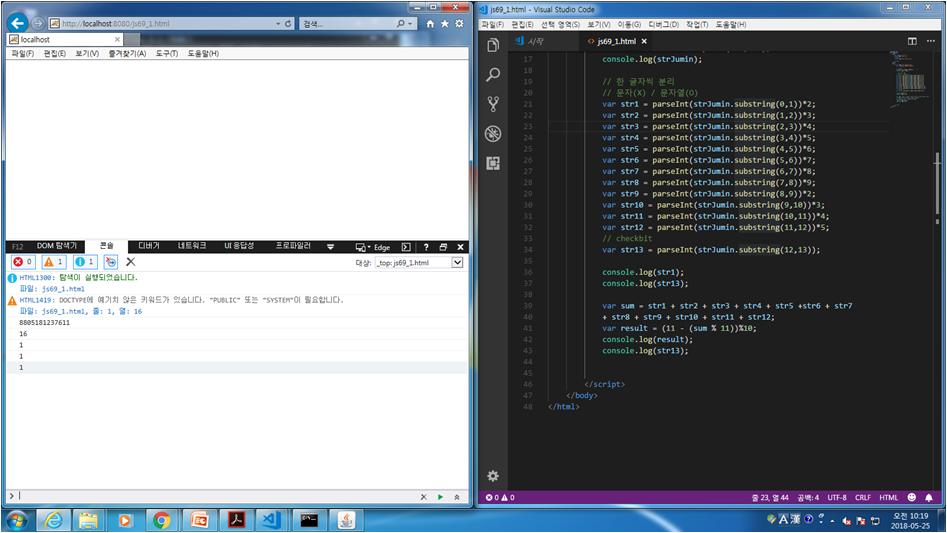
js.69_2 반복 소스 loop화
<!doctype html />
<html lang="ko">
<head>
<meta charset="utf-8"/>
<style type="text/css">
</style>
<script type="text/javascript">
</script>
</head>
<body>
<script type="text/javascript">
var strJumin="880518-1237611";
strJumin = strJumin.replace('-', '');
var bits = [2, 3, 4, 5, 6, 7, 8, 9, 2, 3, 4, 5];
var sum = 0;
for(var i=0; i<bits.length; i++) {
sum += parseInt(strJumin.substring(i, i+1)) * bits[i];
}
var last = parseInt(strJumin.substring(12, 13));
var result = (11 - (sum % 11)) % 10;
console.log(last);
console.log(result);
</script>
</body>
</html>

js.69_3 함수화
<!doctype html />
<html lang="ko">
<head>
<meta charset="utf-8"/>
<style type="text/css">
</style>
<script type="text/javascript">
var checkJumin = function(strJumin) {
strJumin = strJumin.replace('-', '');
var bits = [2, 3, 4, 5, 6, 7, 8, 9, 2, 3, 4, 5];
var sum = 0;
for(var i=0; i<bits.length; i++) {
sum += parseInt(strJumin.substring(i, i+1)) * bits[i];
}
var last = parseInt(strJumin.substring(12, 13));
var result = (11 - (sum % 11)) % 10;
if(last == result) {
return '맞음';
}
else {
return '틀림';
}
}
</script>
</head>
<body>
<script type="text/javascript">
var result = checkJumin('880518-1237611');
console.log(result);
</script>
</body>
</html>

'Web & Mobile > Javascript' 카테고리의 다른 글
Lecture 10 - Javascript(6) (0) | 2019.04.14 |
---|---|
Lecture 9 - Javascript(5) (0) | 2019.04.14 |
Lecture 7 - Javascript(3) (0) | 2019.04.13 |
Lecture 6 - Javascript(2) (0) | 2019.04.13 |
Lecture 5 - Javascript(1) (0) | 2019.04.13 |
댓글